Session saving and Firefox 1.5
I use mainly Firefox 1.5 as internet browser, and my friends call me "the thousand tabs man" for joke because I often have many tabs open. So, it's important for me to keep these tabs even when I close my pc or when I experience a firefox crash.
So I tried to look for an extension that provides this feature, and I installed SessionSaver 2. All ok with old Firefox < 1.5, but with the latest version I get some problems: the worst one is the "unresponsive script" warning that I get during operation such as file uploads, even when the file is small. It seems as the SessionSaver script computes some operations that stick it in an infinite loop or something similar, because if I click on "stop script" button the upload completes correctly.
I removed SessionSaver extension, and asked in the official forum (it's not properly a forum, is a post on mozillazine forum with actual 122 pages, not very well organized in my opinion), and found there a link to another extension: TabMixPlus.
The image on the left shows you part of session saving options you can choose. It has also a lot of other useful features, such as multi level undo close tab - I used to install a separate extension for this, called UndoCloseTab - and page loading progress bar on the tab and, if you want, in statusbar too. I still have to explore all its features, but the only one I really needed works perfectly, and I have my tab session always present even in case of system crash.
You can download TabMixPlus here:
http://tmp.garyr.net/beta%200.3/
Official MozillaZine thread about TabMixPlus is here:
http://forums.mozillazine.org/viewtopic.php?t=327222
It's still a beta version, but it seems to work well. Many thanks to the author(s).
So I tried to look for an extension that provides this feature, and I installed SessionSaver 2. All ok with old Firefox < 1.5, but with the latest version I get some problems: the worst one is the "unresponsive script" warning that I get during operation such as file uploads, even when the file is small. It seems as the SessionSaver script computes some operations that stick it in an infinite loop or something similar, because if I click on "stop script" button the upload completes correctly.
I removed SessionSaver extension, and asked in the official forum (it's not properly a forum, is a post on mozillazine forum with actual 122 pages, not very well organized in my opinion), and found there a link to another extension: TabMixPlus.
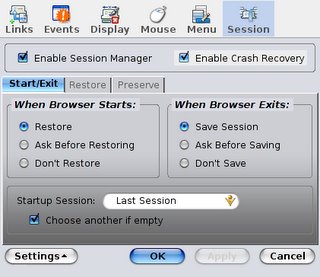
You can download TabMixPlus here:
http://tmp.garyr.net/beta%200.3/
Official MozillaZine thread about TabMixPlus is here:
http://forums.mozillazine.org/viewtopic.php?t=327222
It's still a beta version, but it seems to work well. Many thanks to the author(s).